Java Instantiate
To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation.
Java instantiate. Type in the following Java statements:. In java linkedlist is a collection class that can be used both as a queue as well as a list. How to use instantiate in a sentence.
Creating new instances of objects to be used in a program. This text will get into more detail about the Java Constructor object. Sometimes you want to create a Java class that can’t be instantiated at all.
Please suggest how can I achieve the same. This may seem a trivial Generics Problem. Java classes consist of variables and methods (also known as instance members).
The Scanner class is used to get user input, and it is found in the java.util package. Enums are for when you have a fixed set of related constants. Instantiate definition is - to represent (an abstraction) by a concrete instance.
So, if you have an interface called SomeInterface, then the following code will never compile:. They are called so because their values are instance specific and are not shared among instances. These variables are initialized when the class is instantiated.
An instantiated object is given a name and created in memory or on disk using the structure described within a class declaration. To instantiate a Date object, or any other object, use the new operator. If you try to do so, a compile time error will be generated.
The return value is either true or false. No, you cannot instantiate enums, and there's a good reason for that. Asked Oct 21, 19 in Big Data Hadoop & Spark by Kartik (11.9k points) I have Hadoop 2.7.1 and apache-hive-1.2.1 versions installed on ubuntu 14.0.
What is instance variable in Java?. If the inner class is public & the containing class as well, then code in some other unrelated class can as well create an instance of the inner class. SCJP 1.4 - SCJP 6 - SCWCD 5 - OCEEJBD 6 - OCEJPAD 6 How To Ask Questions How To Answer Questions.
In the most general sense, you create a thread by instantiating an object of type Thread. Java Reflection provides ability to inspect and modify the runtime behavior of application. The instance being a specific object rather than the idea of it;.
An instance (predicate logic), a statement produced by applying universal. The instanceof keyword compares the instance with type. Here, I instantiate objects within the main class, calling the Account class.
Instance variables − Instance variables are variables within a class but outside any method. For example, the definition of a generic list class OrderedList<T> might require the element type T to be a subtype of the interface Comparable<T> 1 :. An instance variable belongs to a class.
Abstract classes are similar to interfaces. You may need to create similar classes …. Java instanceof and its applications Last Updated:.
1) In object-oriented programming, some writers say that you instantiate a class to create an object, a concrete instance of the class. Instantiation is a big word to describe a universal and straightforward concept in Java programming:. Actually this line is sufficient to create a Java object.
And that method parameter will accept – as an. New requires a single argument:. Declarations do not instantiate objects.
"Cannot instantiate Java class" WcmInbox. Then, i tried to dig down what is happening actually. SomeInterface s = new SomeInterface( );.
However, because an interface is a type, you are allowed to write a method with a parameter of an interface type. Java generics why this won’t work Duplicate:. To provide an instance of or concrete evidence in support of (a theory, concept, claim, or the like).
Let's take a sample app that needs to use a Bar object. Hi, I searched the problem from the discussion board and I noticed that similar errors has been occured, however, I didn't find the solution for Documentum 6.5. Definition and Usage The instanceof keyword checks whether an object is an instance of a specific class or an interface.
But, DO NOT instantiate it. The java instanceof operator is used to test whether the object is an instance of the specified type (class or subclass or interface). This lesson will define the term and.
If a class has an instance variable, then a new instance variable is created. Its methods provide utility-type functions that aren’t really associated with a particular object. Using Java Reflection you can inspect the constructors of classes and instantiate objects at runtime.
Instance variables in Java are non-static variables which are defined in a class outside any method, constructor or a block. These methods do not create a prefab connection to the new instantiated object. Java variables are two types either primitive types or reference types.
Since the type parameter not class or, array, You cannot instantiate it. Instantiating Prefabs at run time PrefabUtility.InstantiatePrefab. A slightly more complex example where the generated class also implements java.lang.Runnable (runnable objects are commonly used with threads and java.util.concurrent classes) which defines one.
You can extend the Thread class ;. Because Java supports separate class compilation, the only constraint on instantiating C<T> in client code is the upper bound, if any, declared for T in the definition of C<T>. Open your text editor and create a new file.
The constructor method is responsible for initializing the new object. - Okay, now comes the fun part.…Let's return to our Payroll application.…We have created the employee and the address classes…so let's take a look at the main class,…which I have open here, payroll.java.…You'll notice line 10 has "public static void main".…In this class, we're going to be able to create…objects for the address and the employee.…We're then going to print the. The instanceof in java is also known as type comparison operator because it compares the instance with type.
First, let us discuss how to declare a class, variables and methods then we will discuss access modifiers. A call to a constructor. The instantiation principle, the idea that in order for a property to exist, it must be had by some object or substance;.
You must be wondering about what exactly is an Instance. When you create an object, you are creating an "instance" of a class, therefore "instantiating" a class. Both instantiate the single.
Now if you want to re-use this object somewhere, you need to decare it with the appropriate type. Write a static/factory method that checks the static instance member for null and creates the. Unable to instantiate org.apache.hadoop.hive.ql.metadata.SessionHiveMetaStoreClient.
To invoke a instance method, we have to create an Object of the class in within which it defined. 10-10-19 instanceof is a keyword that is used for checking if a reference variable is containing a given type of object reference or not. Instantiating an Object The new operator instantiates a new object by allocating memory for it.
To instantiate is to create such an instance by, for example, defining one particular variation of object within a class, giving it a name, and locating it in some physical place. Instance variable in Java is used by Objects to store their states. Java Download » What is Java?.
In·stan·ti·at·ed , in·stan·ti·at·ing , in·stan·ti·ates To represent by a concrete or tangible example:. The Constructor class is obtained from the Class object. Using java reflection we can inspect a class, interface, enum, get their structure, methods and fields information at runtime even though class is not accessible at compile time.We can also use reflection to instantiate an object, invoke it.
If you really want to write your enum like the way you did, then you can make the toString method static, so. See the Theory of Forms;. The idea is to use interfaces and Java reflection to allow your application to instantiate certain classes based on runtime configuration, instead of hard-coding instantiation of those classes.
» Uninstall About Java. You don't want to instantiate one, because then the set would not be fixed. Static methods vs Instance methods in Java Last Updated:.
Queue is an interface, you can’t explicitly construct a Queue, you’ll have to instantiate one of its implementing classes. The lazy approach to instantiating a Singleton is unnecessary in Java because of the way in which the Java runtime handles class loading and static instance variable initialization. Each instantiated object of the class has a separate copy or instance of that variable.
The outer class (the class containing the inner class) can instantiate as many numbers of inner class objects as it wishes, inside its code. You can use them as reference types or return types, but the actual value must be the instance of a non-abstract class. Here is an example:.
Here’s a link to the Java tutorial on this subject. You cannot instantiate them, and they may contain a mix of methods declared with or without an implementation. Introduction:in this blog we will learn about linkedlist.in general terms, linkedlist is a data structure where each element consist of three parts.
In the following Java example we have created a class of generic type named Student where, T is the generic parameter later in the program we are trying to instantiate this parameter using the new keyword. Below is my autopsy report:. One day i saw a very strange thing while coding in java that i can instantiate interfaces.
Creating objects with a prefab connection can be achieved using PrefabUtility.InstantiatePrefab. This is done via the Java class java.lang.reflect.Constructor. Cannot instantiate the type?.
The new operator requires a single, postfix argument:. Reflection in Java is one of the advance topic of core java. Instance variables can be accessed from inside any method, constructor or blocks of that particular class.
Java defines two ways in which this can be accomplished:. 🙂 suppose I have the class declaration. In our example, we will use the nextLine() method, which is used to read Strings:.
Variables that are defined without the STATIC keyword and are Outside any method declaration are Object-specific and are known as instance variables. A constructor method for the object to be created. The object you have instantiated is referred to as person.
You can implement the Runnable interface. Instantiating a generic class in Java I would like to create an object of Generics Type in java. Queue linkedList = new LinkedList();.
Instance method are methods which require an object of its class to be created before it can be called. Instantiate synonyms, instantiate pronunciation, instantiate translation, English dictionary definition of instantiate. A modern concept similar to participation in classical Platonism;.
No objects have been instantiated yet, so the reference variables a , b , and c do not refer to any objects. Following is a Java program to show different behaviors of instanceof. Make a private static instance (class-member) of this singleton class.
Verb (used with object), in·stan·ti·at·ed, in·stan·ti·at·ing. However, with abstract classes, you can declare fields that are not static and final, and define public, protected, and private concrete methods. First part represents the link to the previous element, second part represents the value of the element and last one represents the next element.
The name of the constructor provides the name of the class to instantiate. A good example in the Java API is the Math class. The easiest way to create a thread is to create a class that implements the Runnable interface.
This video continues from Tutorial 14. Or as you wrote it Person person = new. In C++ and other similar languages, to instantiate a class is to create an object, whereas in Java, to instantiate a class creates a specific class.
October 15, 08 edited January 23, 09 in Documentum. No, an interface can not be instantiated in Java. Such a class consists entirely of static fields and methods.
Instantiating the Point Objects Here is a picture of the variables just as the program starts running. To instantiate an object in Java, follow these seven steps. Clojure compiler will generate an anonymous class for this proxy and at runtime, the cost of a proxy call is the cost of instantiating this class (the class is not generated anew on every single call).
Person = new Person();. It returns either true or false.

Engine Exception While Instantiating Class Engine Cannot Load Class Cockpit Tasklist Admin Web Camunda Bpm Forum

Pimeta Instantiation Extract For Java Download Scientific Diagram
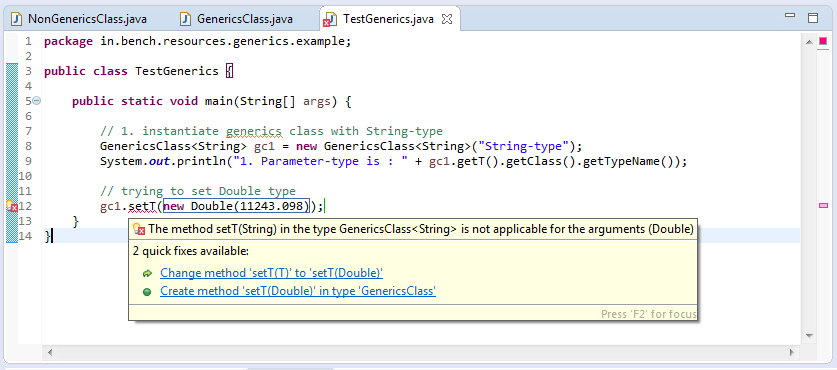
Generics Classes In Java Benchresources Net
Java Instantiate のギャラリー

Programming Guide For Lambari And Pacu Versions
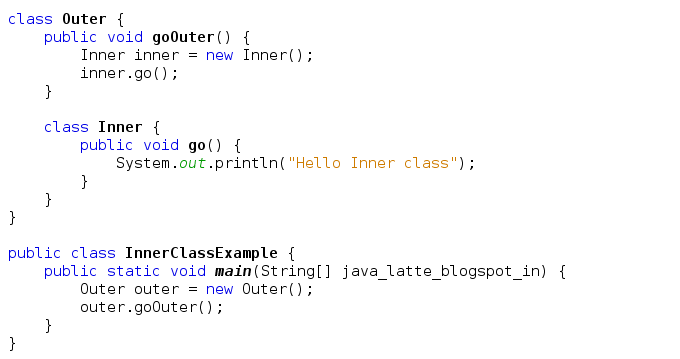
Flavors Of Nested Classes In Java 8 Laptrinhx

How Do I Instantiate An Object Of A Class Via Its String Name Web Tutorials Avajava Com

Quiz Worksheet Instantiation In Java Study Com

Java List How To Create Initialize Use List In Java

Bean Instantiation Order Cuba Platform
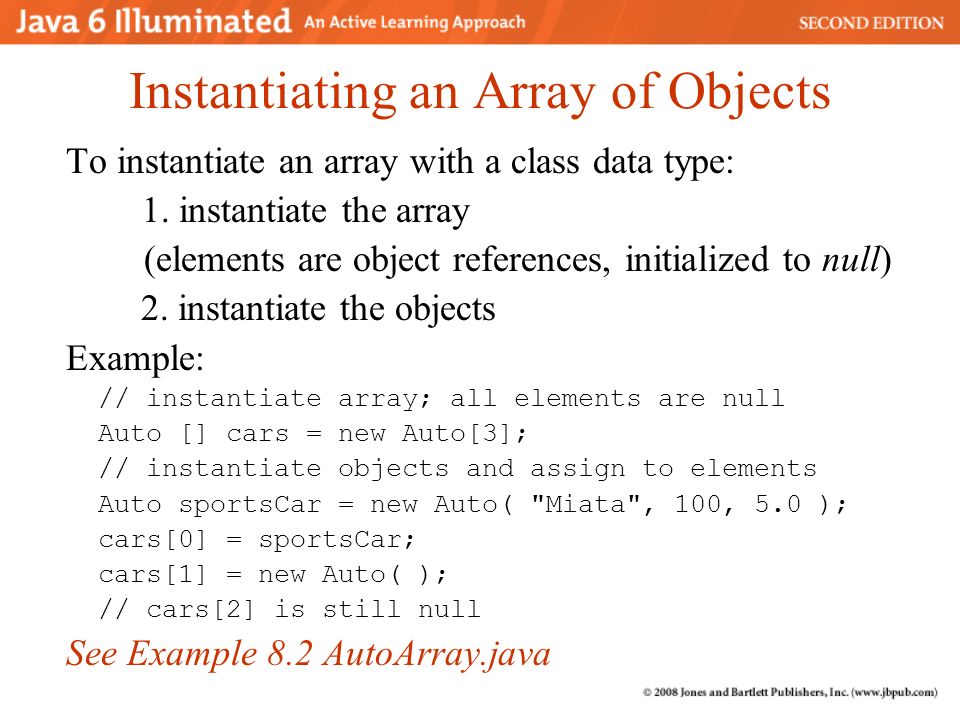
Chapter 8 Single Dimensional Arrays Topics Declaring And Instantiating Arrays Accessing Array Elements Writing Methods Aggregate Array Operations Using Ppt Download
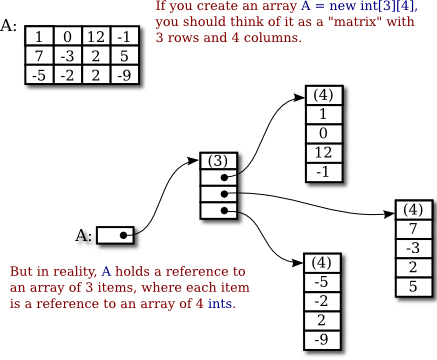
Javanotes 8 1 Section 7 5 Two Dimensional Arrays

Pin On Java Programming Tutorials And Courses
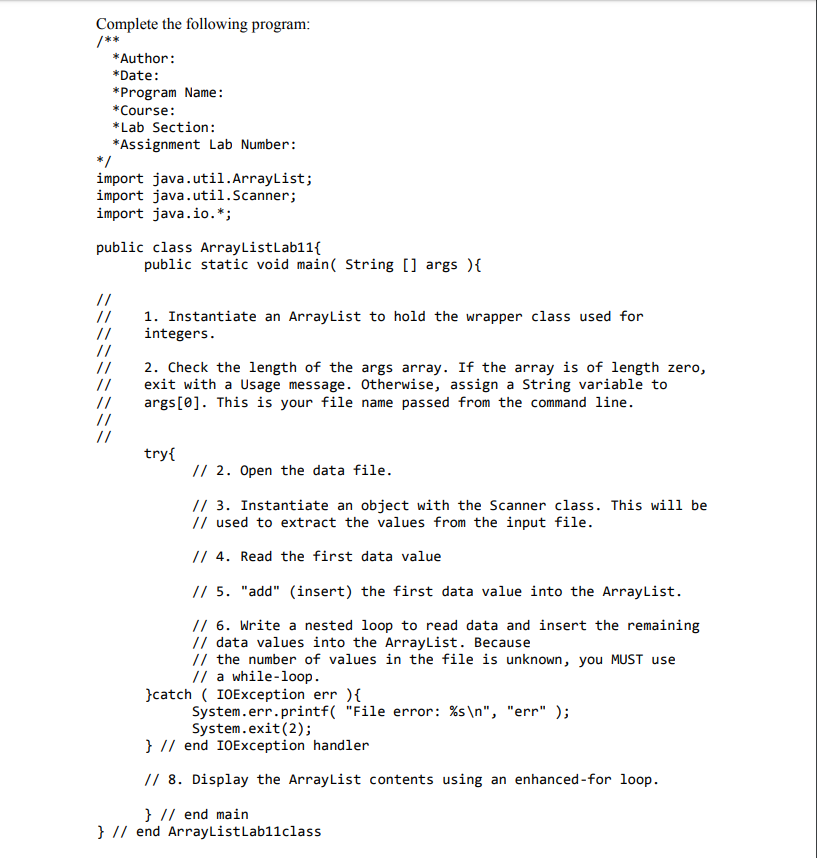
Solved Java The Purpose Of This Assignment Is To Use Chegg Com
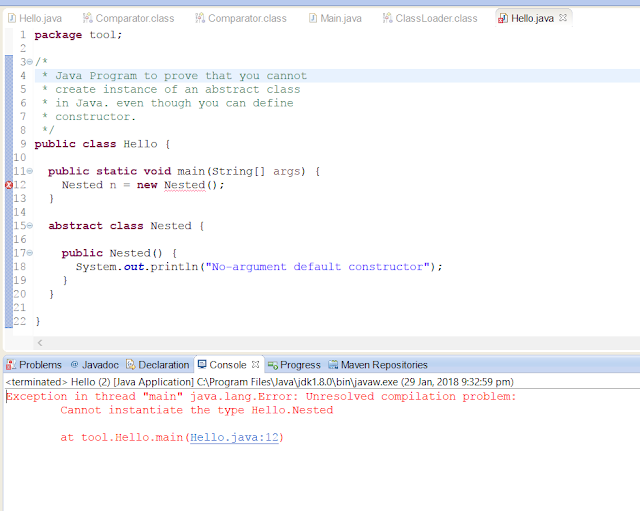
Is It Possible To Create Object Or Instance Of An Abstract Class In Java Java67
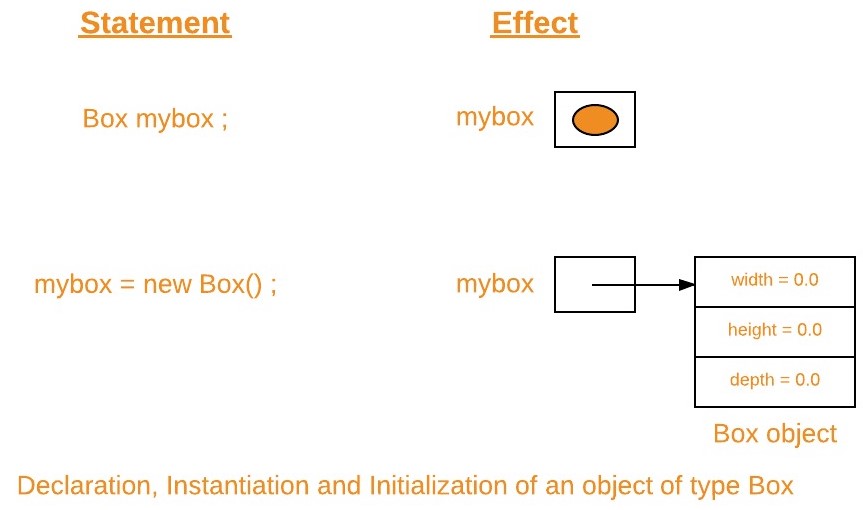
New Operator In Java Geeksforgeeks
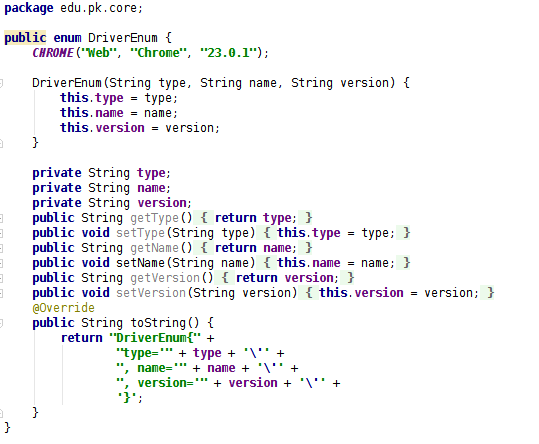
Java In Depth Why Enum Is The Best Approach To Implement A Singleton Class

Must Be Done In Java Provide The Rest Of The Code With Full Comments And Explanation And With Proper Indentation Use Homeworklib
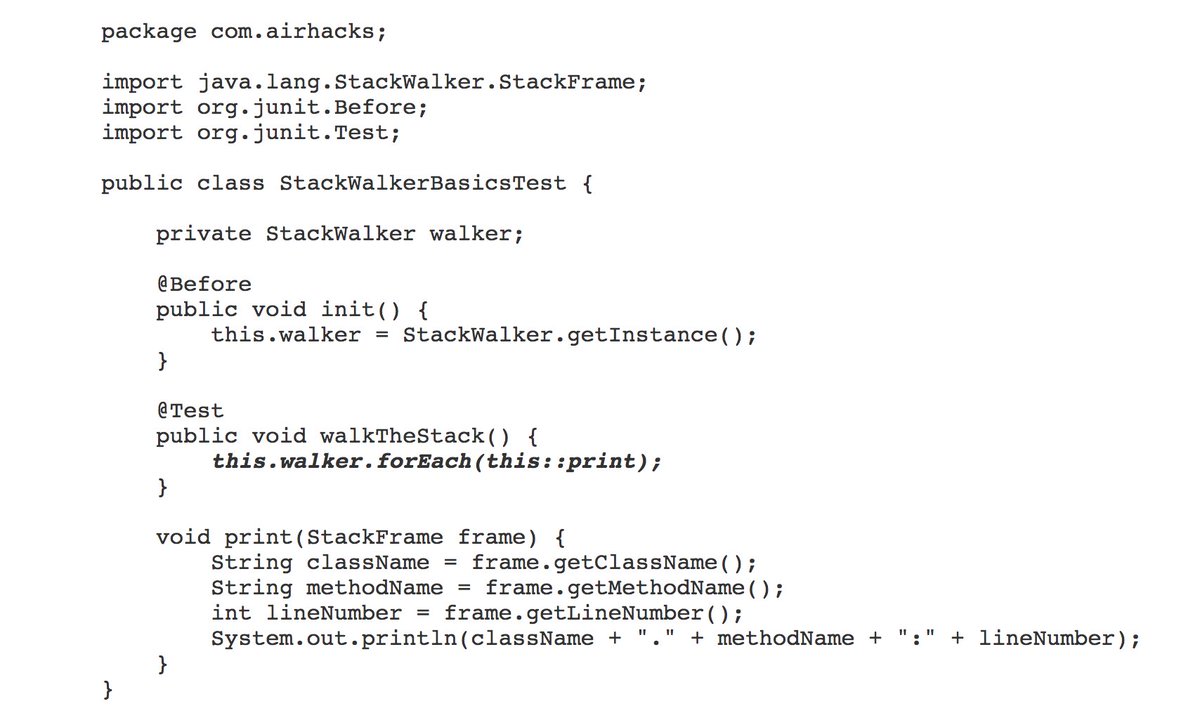
Java Java 9 S Stackwalker Class Allows Stack Walking Without Instantiating An Exception Adambien T Co Rzieiaykot T Co Nev3px49gs

Instantiation Of Placification Of Workspaces For Java C Download Scientific Diagram

Confluence Mobile Ntnu Wiki
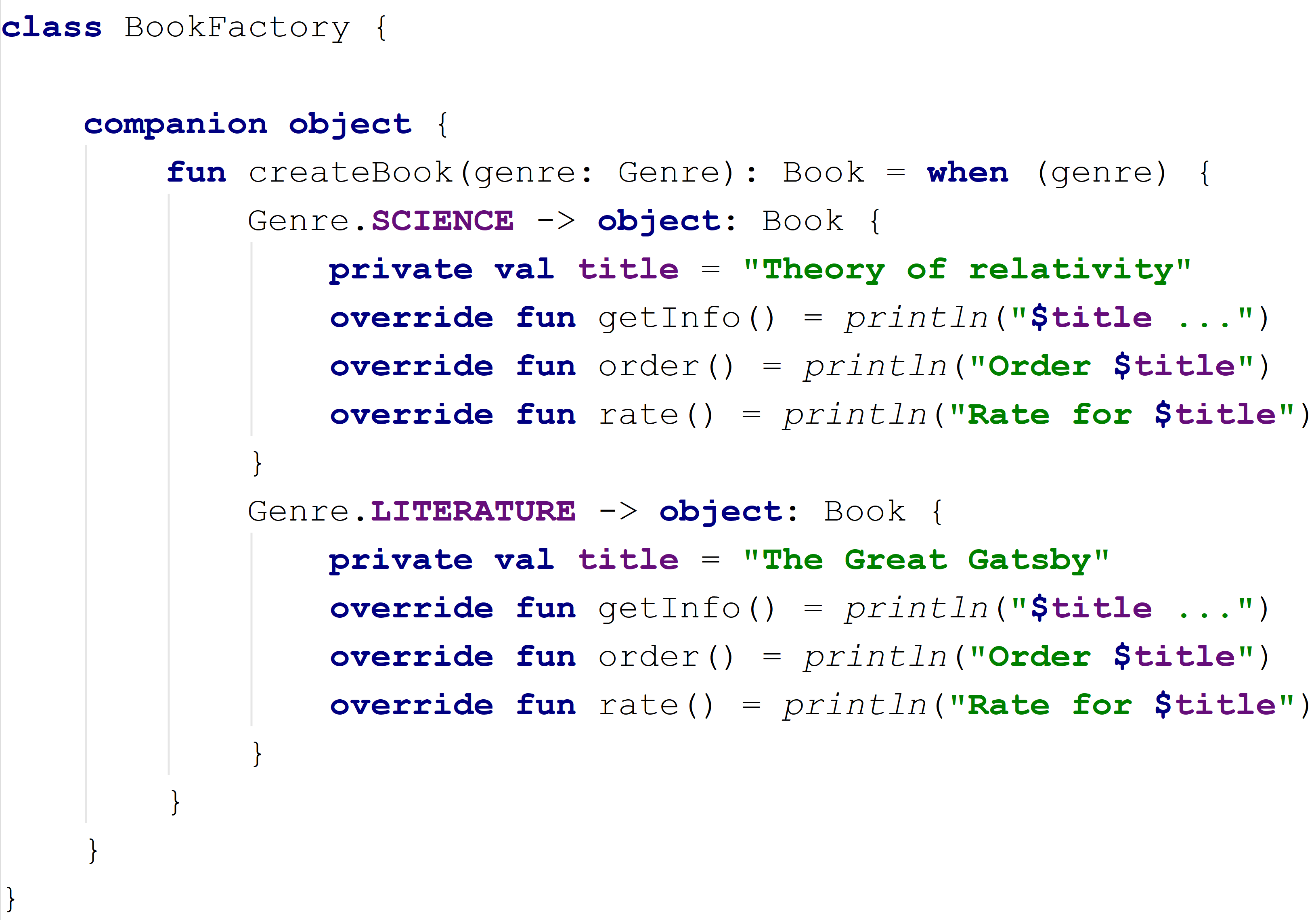
Factory Pattern In Kotlin Dzone Java
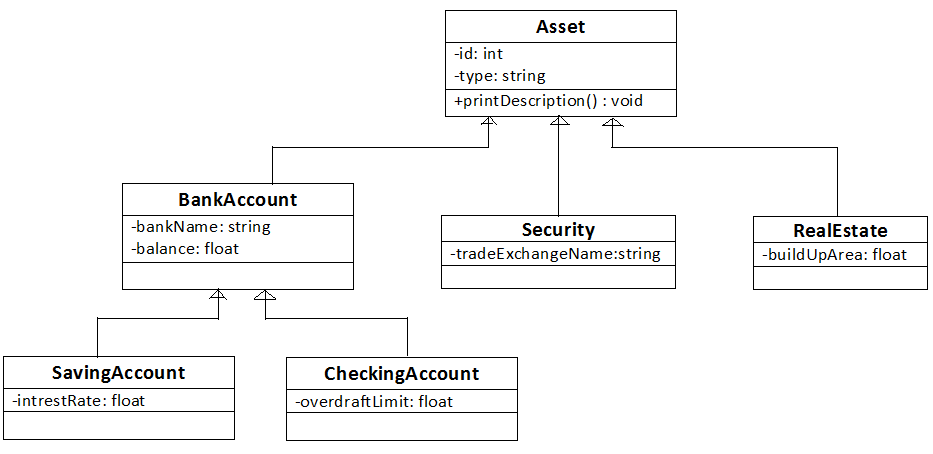
Inheritance In Java Instantiating A Subclass
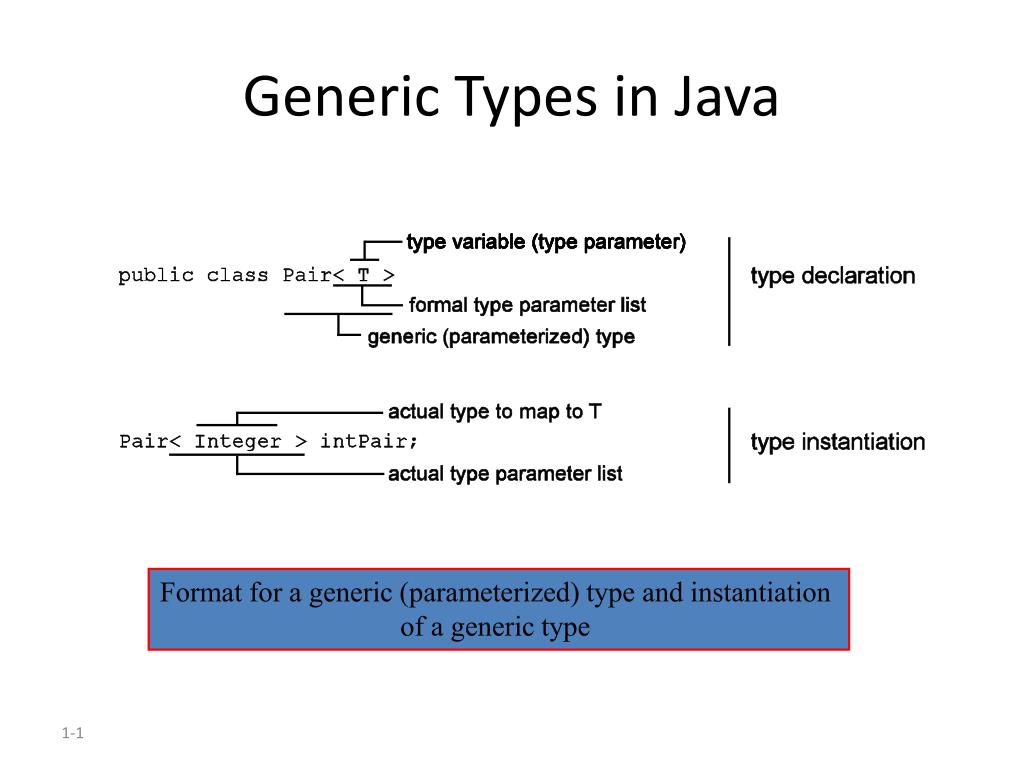
Ppt Generic Types In Java Powerpoint Presentation Free Download Id

How To Instantiate An Object In Java Webucator

Instantiating Classes
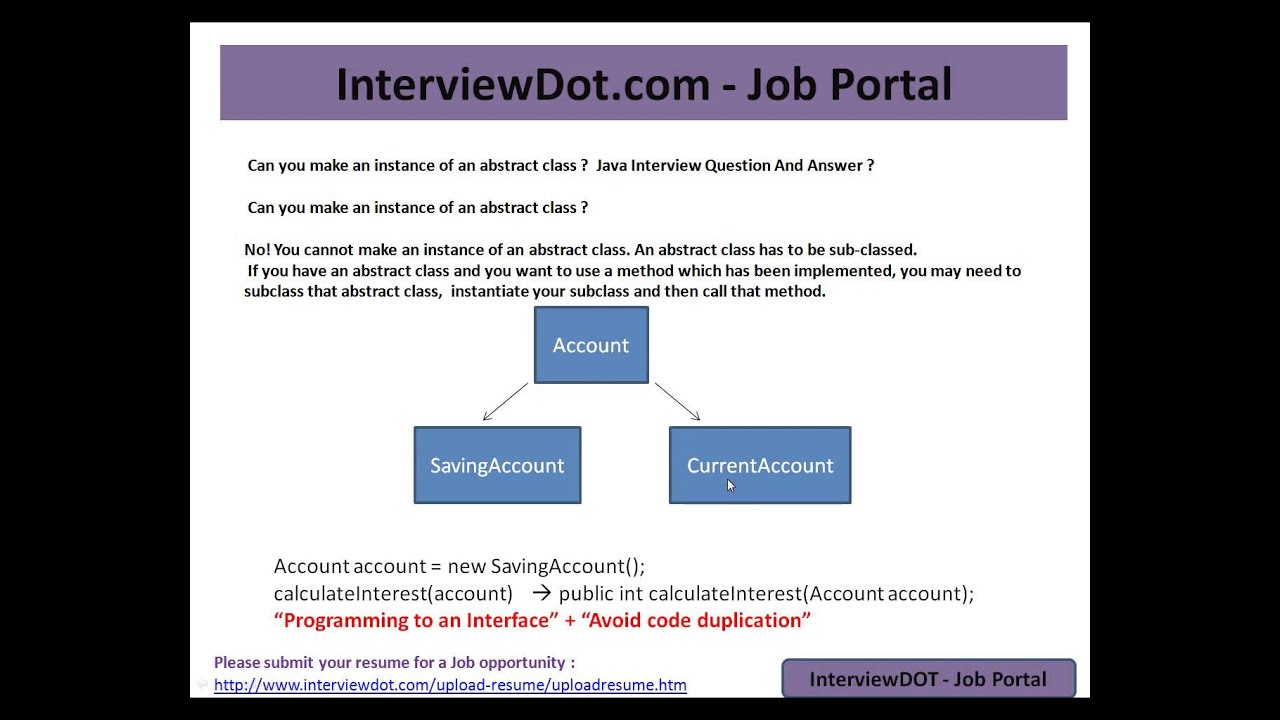
Instantiate An Abstract Class In Java Youtube

Call Java Methods With Dataweave Apisero
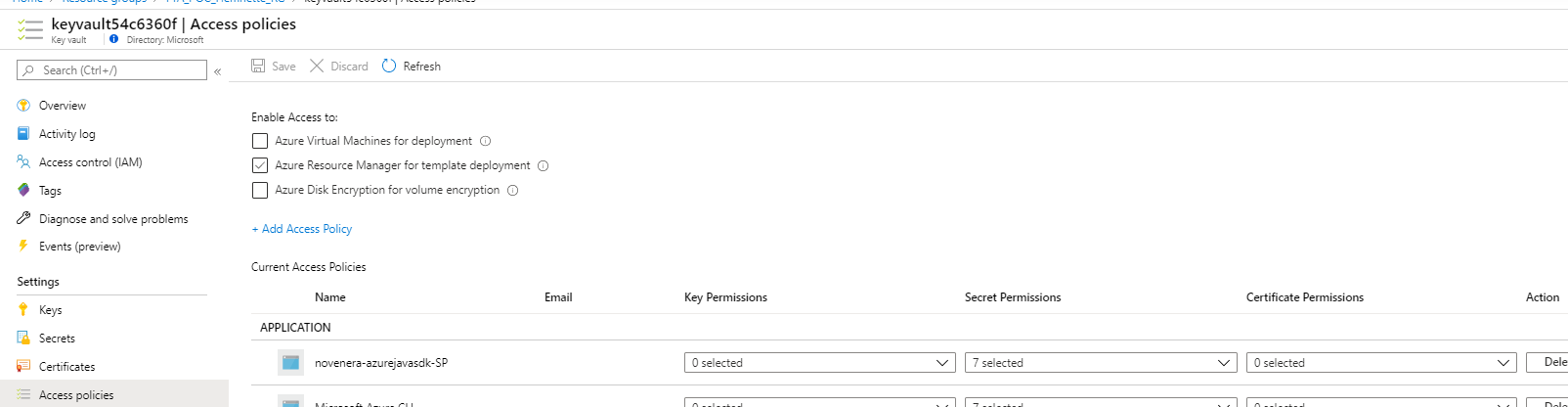
Unable To Instantiate With Defaultazurecredential Despite Setting Required Environment Variables Azure Sdk For Java
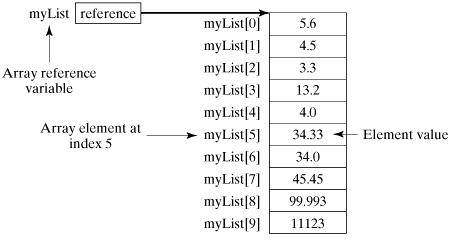
Xyz Code Declare Instantiate Initialize And Use A One Dimensional Array

Cannot Instantiate Class Tests Loginpagetest Software Quality Assurance Testing Stack Exchange

Instantiate A Private Class From Java Main Function Stack Overflow

23 The Instantiation Process Of Class Objects In Java Programmer Sought

This Past Week I Learned Java Object Oriented Programming Polymorphism And Abstraction By Chhaian Pin Medium

Java Class Objects Java Dyclassroom Have Fun Learning
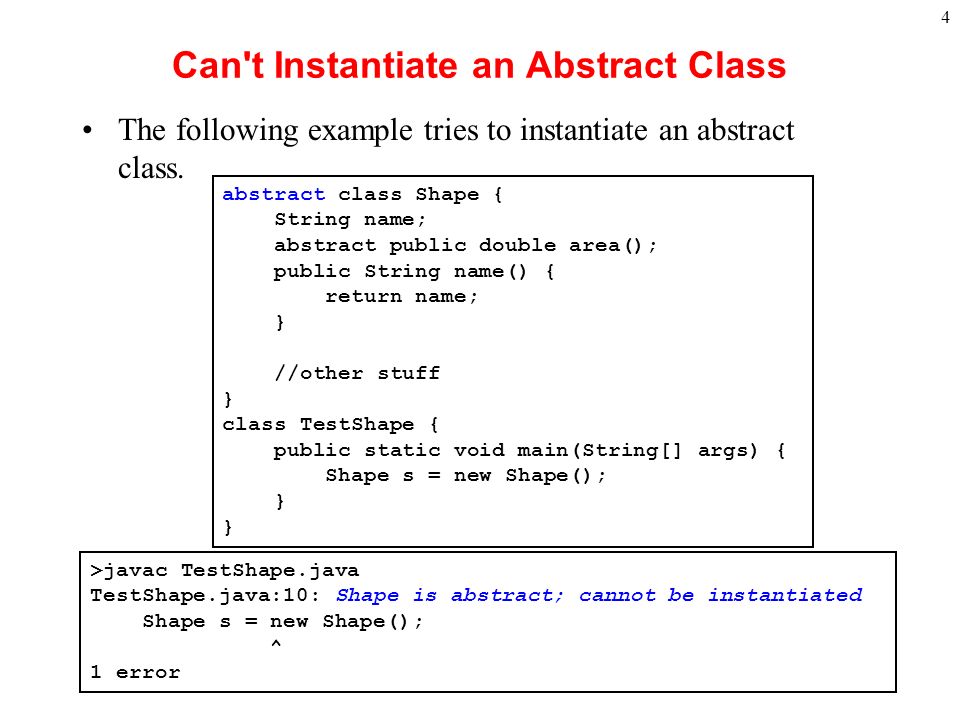
1 Abstract Class There Are Some Situations In Which It Is Useful To Define Base Classes That Are Never Instantiated Such Classes Are Called Abstract Classes Ppt Download
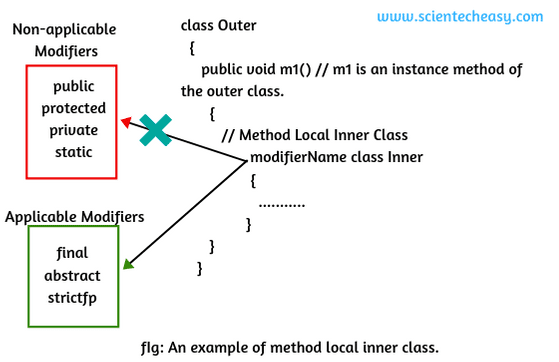
Method Local Inner Class In Java Example Program Scientech Easy
Cannot Instantiate Java Class Dell Community

Programming Guide For Lambari And Pacu Versions
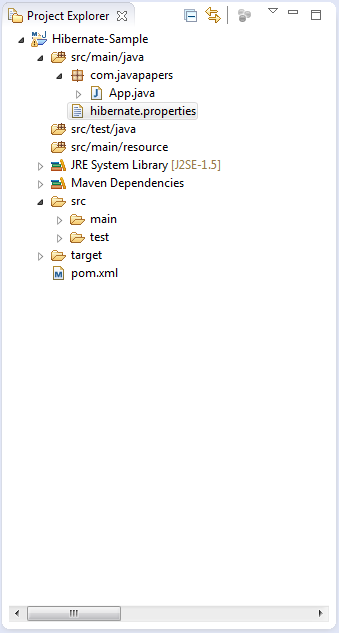
Hibernate Configuration And Sessionfactory Instantiation Javapapers

Oracle Certification Oo Concepts 5 4 Constructors And Instantiation Java 5
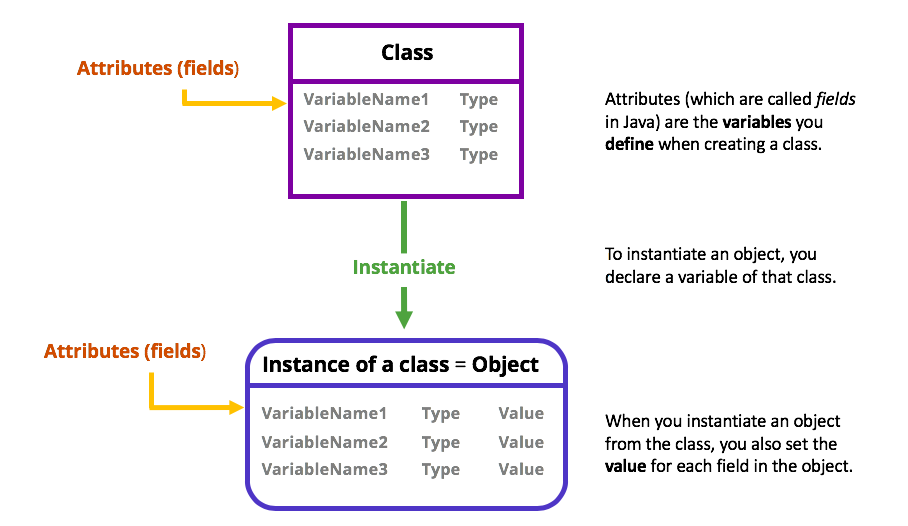
Define Objects And Their Attributes With Classes Learn Programming With Java Openclassrooms
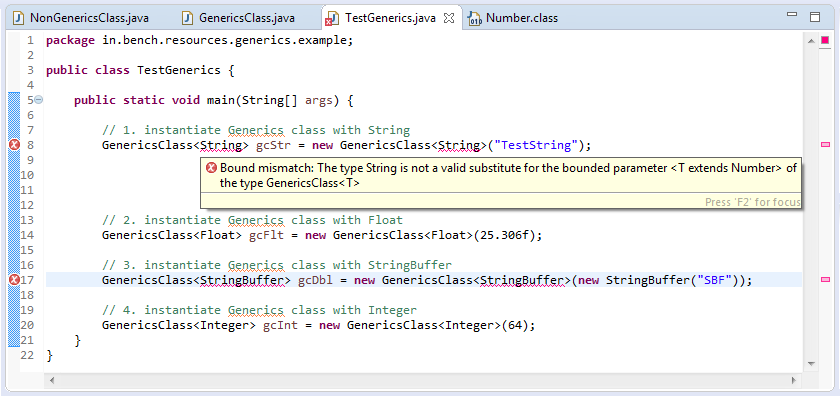
Bounded Types In Generics Benchresources Net
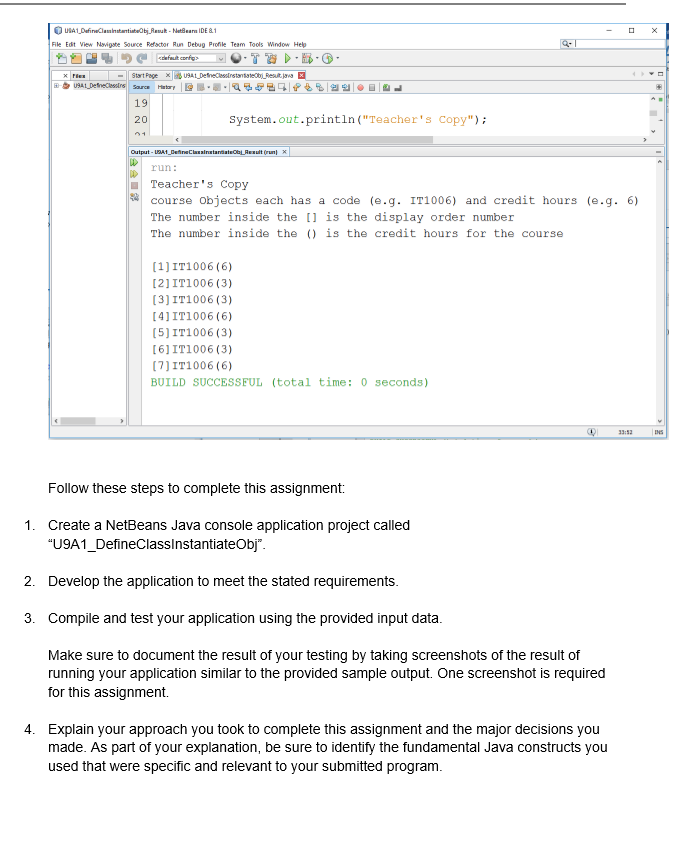
Solved Define Java Classes And Instantiate Their Objects Chegg Com
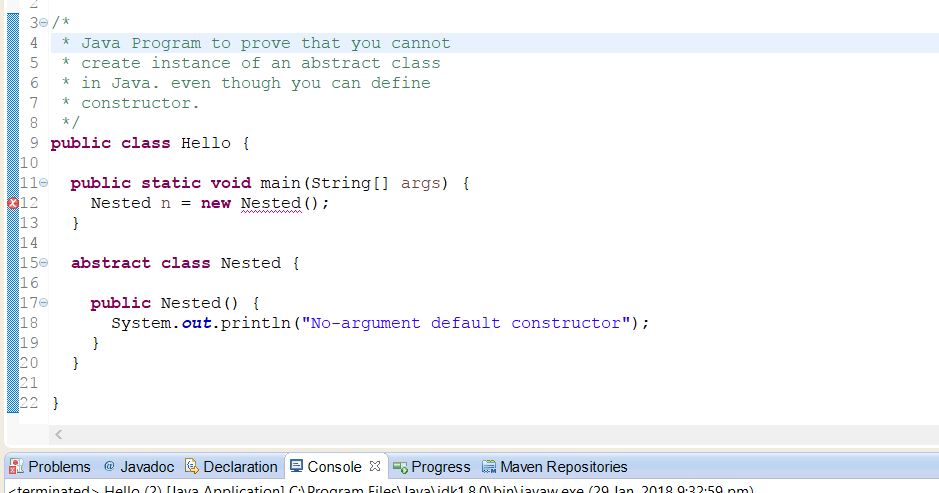
Is It Possible To Create Object Or Instance Of An Abstract Class In Java Java67
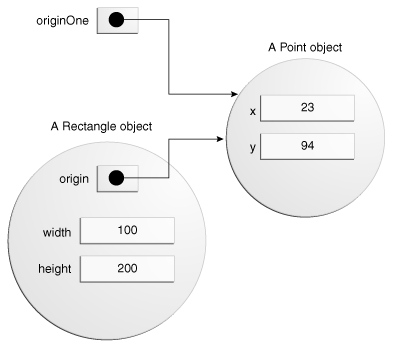
Creating Objects The Java Tutorials Learning The Java Language Classes And Objects

Java Flip Book Pages 301 350 Pubhtml5
Q Tbn 3aand9gctbk7ioze Ygmfb Cxty Ijq1a X3zfktrcvhsso4fnbwwrnn1 Usqp Cau
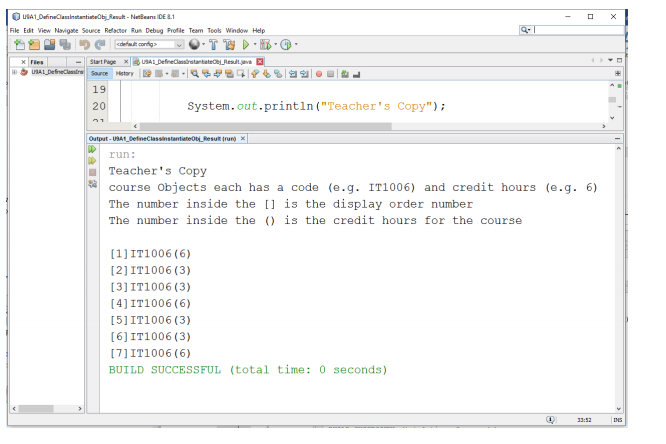
Solved Need Java Code For This Define Java Classes And In Chegg Com

Cannot Instantiate The Type Webdriver Stack Overflow

Creating And Instantiating Custom Classes

Classes Vs Interfaces
Issues Jboss Org Secure Attachment Jaxbcontext Pdf

How To Instantiate An Object In Java Webucator
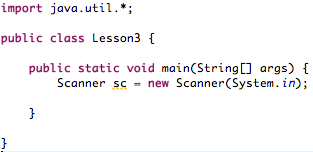
Reading User Input Using The Scanner Class In Java

How To Load And Instantiate Java Class Dynamically Using Java Reflection Api Youtube

Instantiate A Class Software Design And Implementation Past Exam Docsity
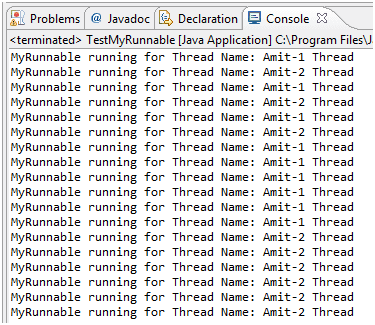
Java Defining Instantiating And Starting Threads W3resource
Tute 3 21 03 09 Integer Computer Science Java Programming Language
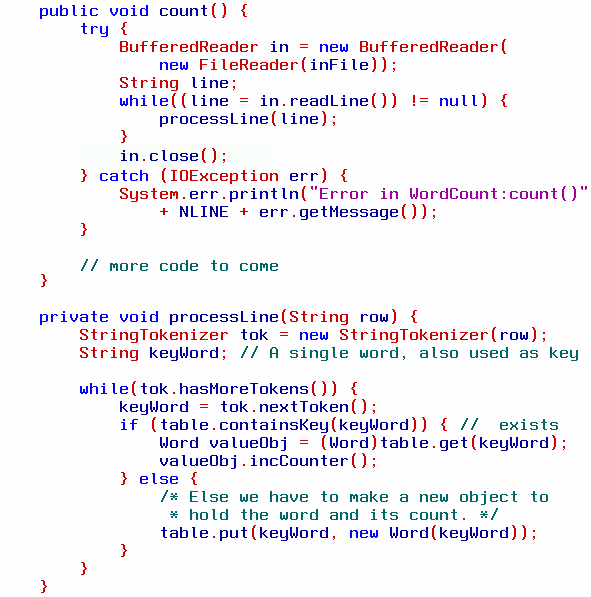
Into Java Part Xiv Edm2
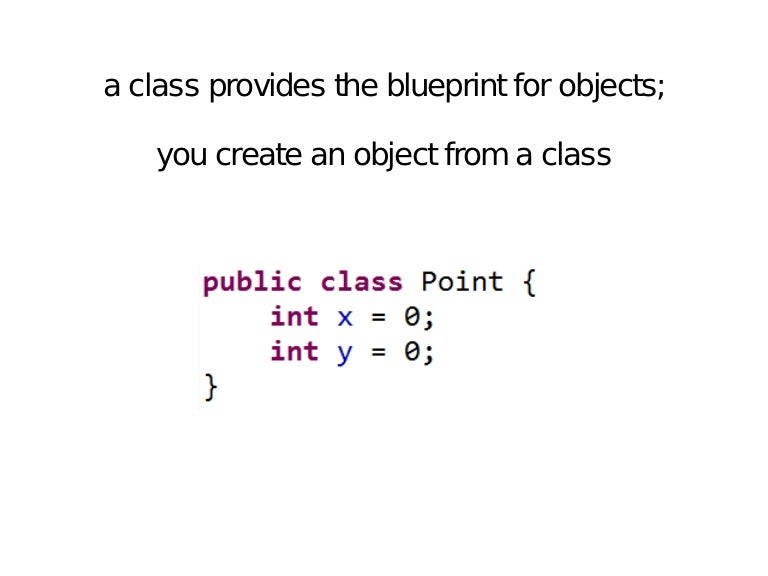
Java Instantiation
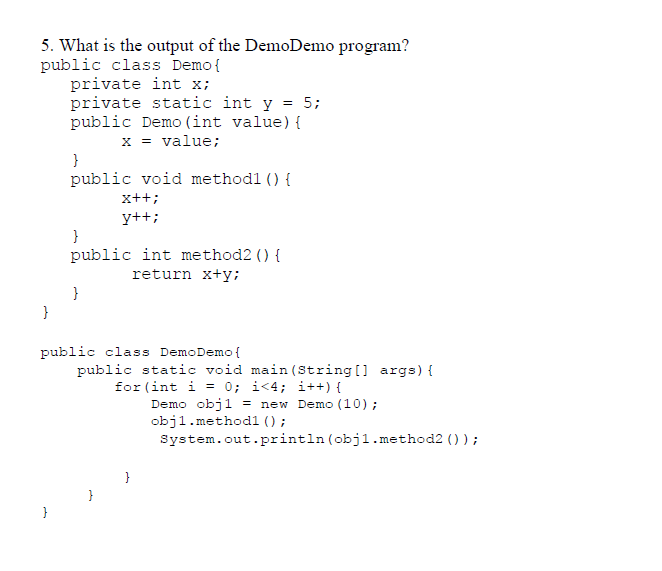
Solved 1 A Class In Java Is Like A B C D A Variable Chegg Com
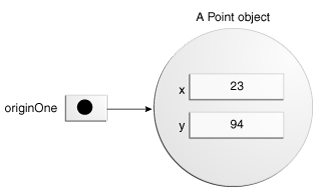
Creating Objects The Java Tutorials Learning The Java Language Classes And Objects

Instantiating Classes Dynamically
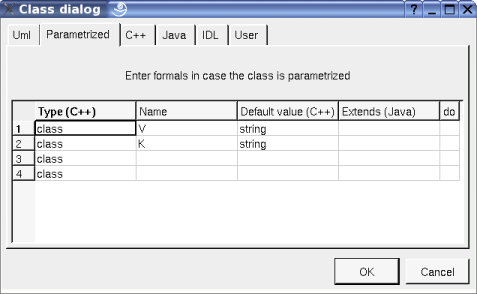
Class

Instantiate Action Error Java Class Not Found Oneplus Community

How Do I Instantiate An Object Of A Class Via Its String Name Web Tutorials Avajava Com

4 The Factory Pattern Baking With Oo Goodness Head First Design Patterns Book

Java Debugger Find Where An Object Was Instantiated Stack Overflow
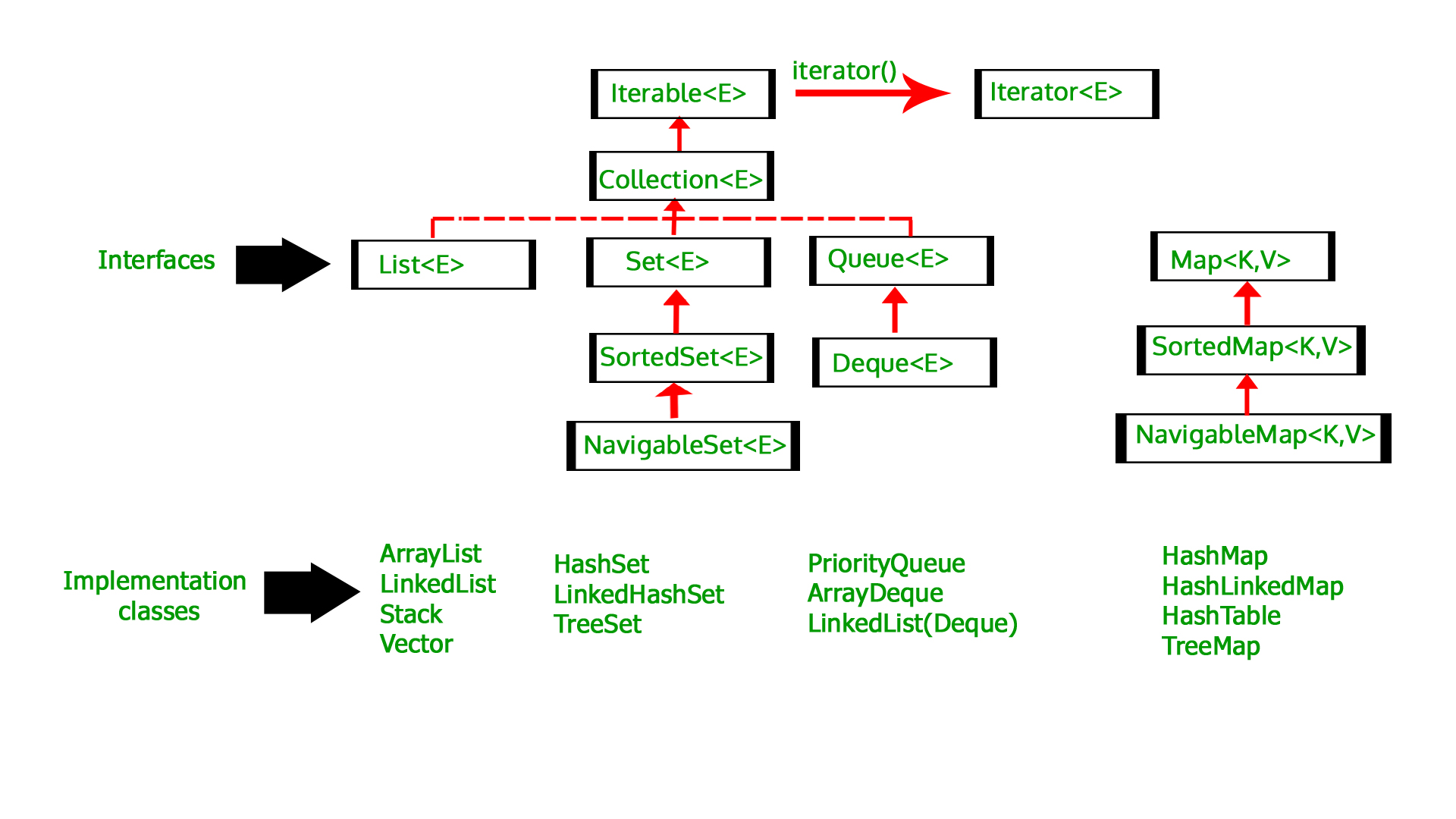
Initialize An Arraylist In Java Geeksforgeeks

Java Generic Arrays Java Tutorial
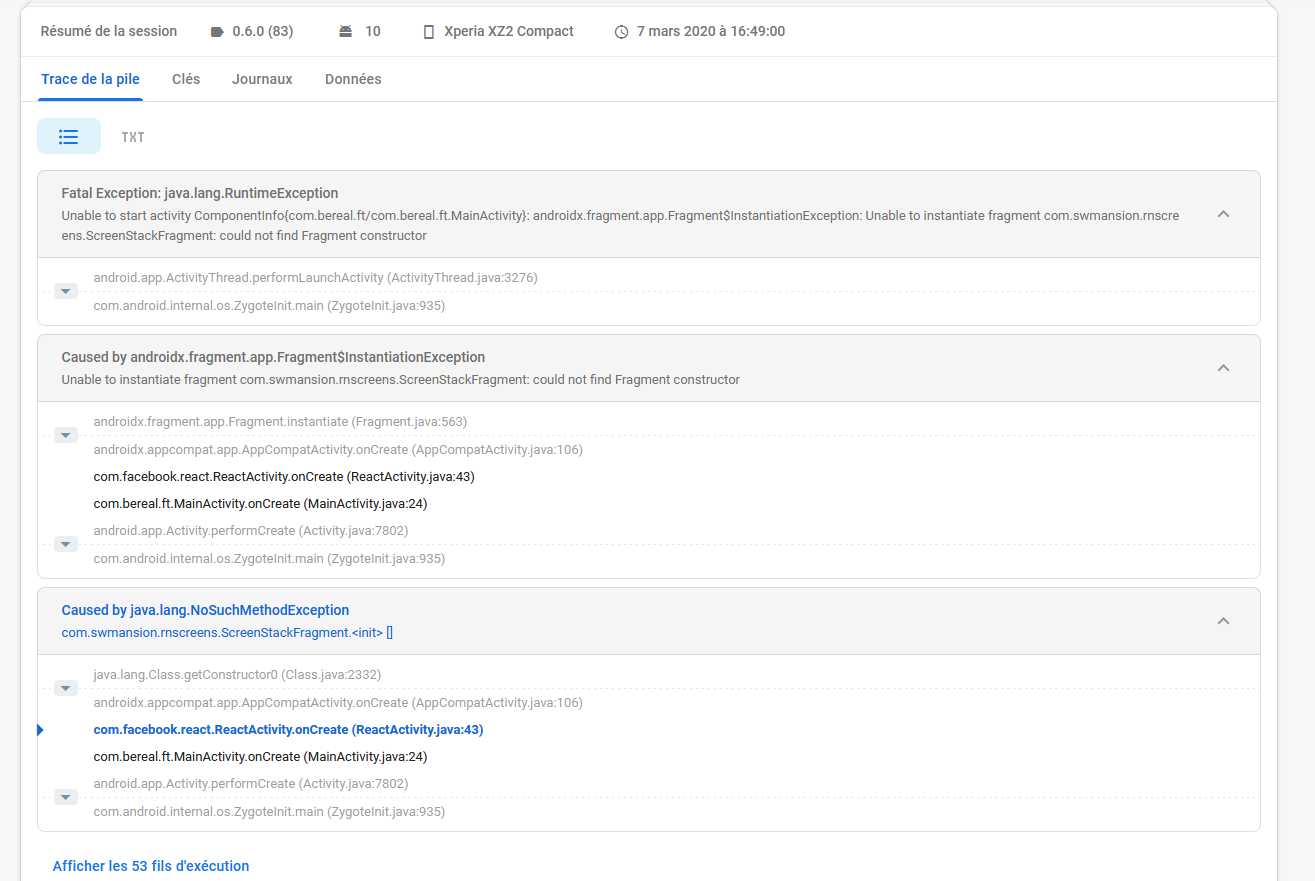
Craslytics Fatal Exception Java Lang Runtimeexception Issue 408 Software Mansion React Native Screens Github

What Is Instantiation In Java Definition Example Video Lesson Transcript Study Com

How To Instantiate An Object In Java Webucator
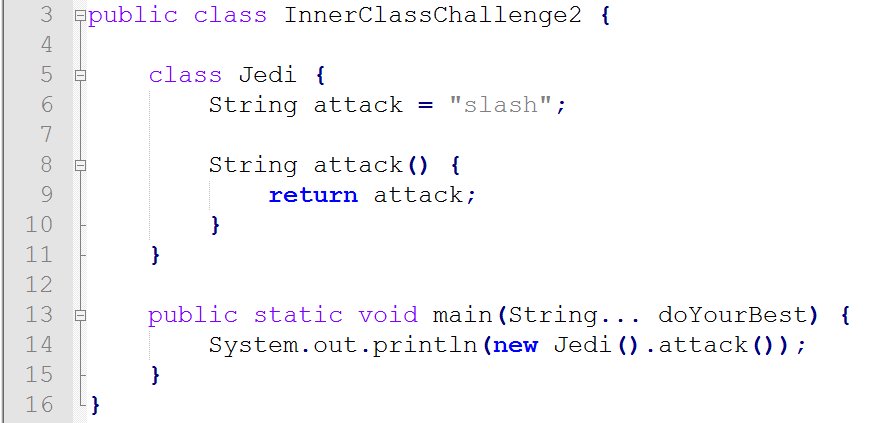
Ina If Want To Reference Attack And Print Slash Make Class Jedi Static Or Instantiate Outer Class First And Then Instantiate Inner One T Co Uo5ksme90v

Java Class Objects Java Dyclassroom Have Fun Learning
Q Tbn 3aand9gctgygpyqeglidm9ymc74huqjchquzeft0vwg1y Isflfytrk1as Usqp Cau

Hiking Around Hackerrank 05 08 01 By Andrew Chen Medium
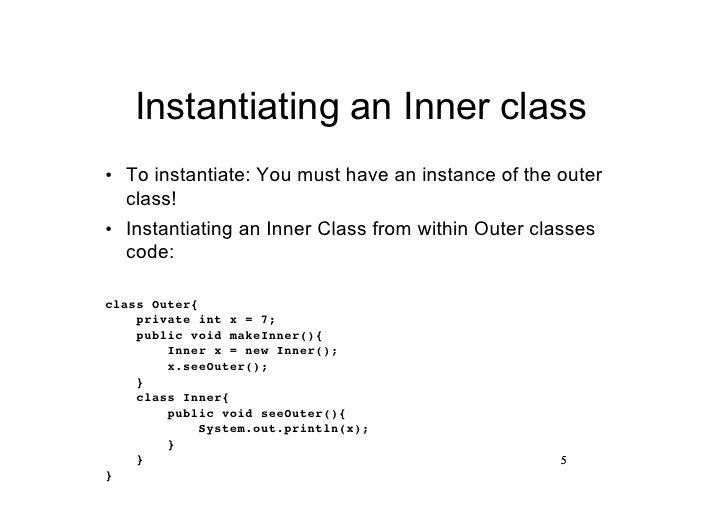
Java Inner Classes

Java Hashmap Inline Initialization Java Tutorial Network

Cannot Instantiate Test S Java Lang Securityexception Prohibited Package Name Java Com Itheima T Programmer Sought

The Process Could Not Be Started Cannot Instantiate Process Definition Modeler Camunda Bpm Forum

When Do I Use New To Instantiate A Class

Do I Need Constructors To Create An Object In Java Quora
Q Tbn 3aand9gctnyfnpuea9rxim2vvka3q3zb14xnm Mxo9qiaaz6voofexe7x8 Usqp Cau
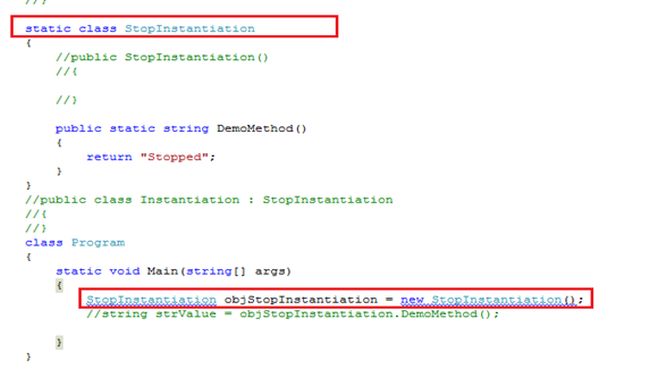
Few Ways To Prevent Instantiation Of Class

Java Chapter 12 Collection Classes
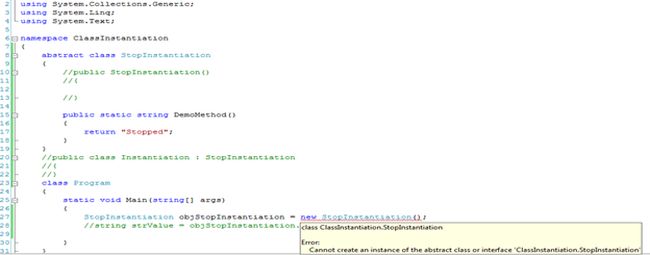
Few Ways To Prevent Instantiation Of Class
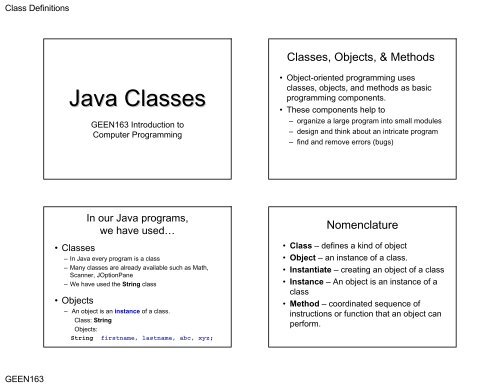
Java Classes

What Is An Abstract Class In Java Talksinfo

Cannot Instantiate The Type Configuration Mirror Api Stack Overflow

How Coldfusion Createobject Really Works With Java Objects
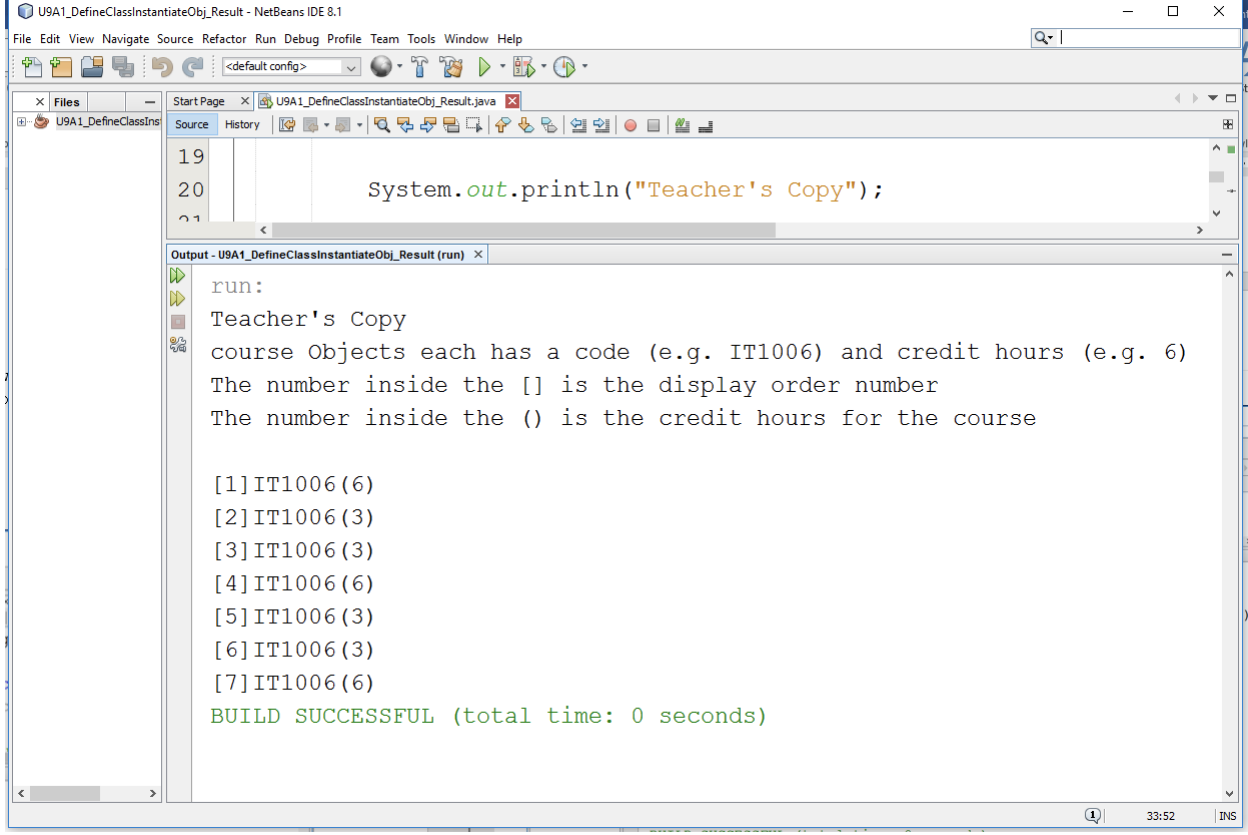
Solved Define Java Classes And Instantiate Their Objects Chegg Com

Cpl79 Y68cbs9m

About The Instantiation Process Of Spring S Bean Object Develop Paper

Abstract Classes And Interfaces
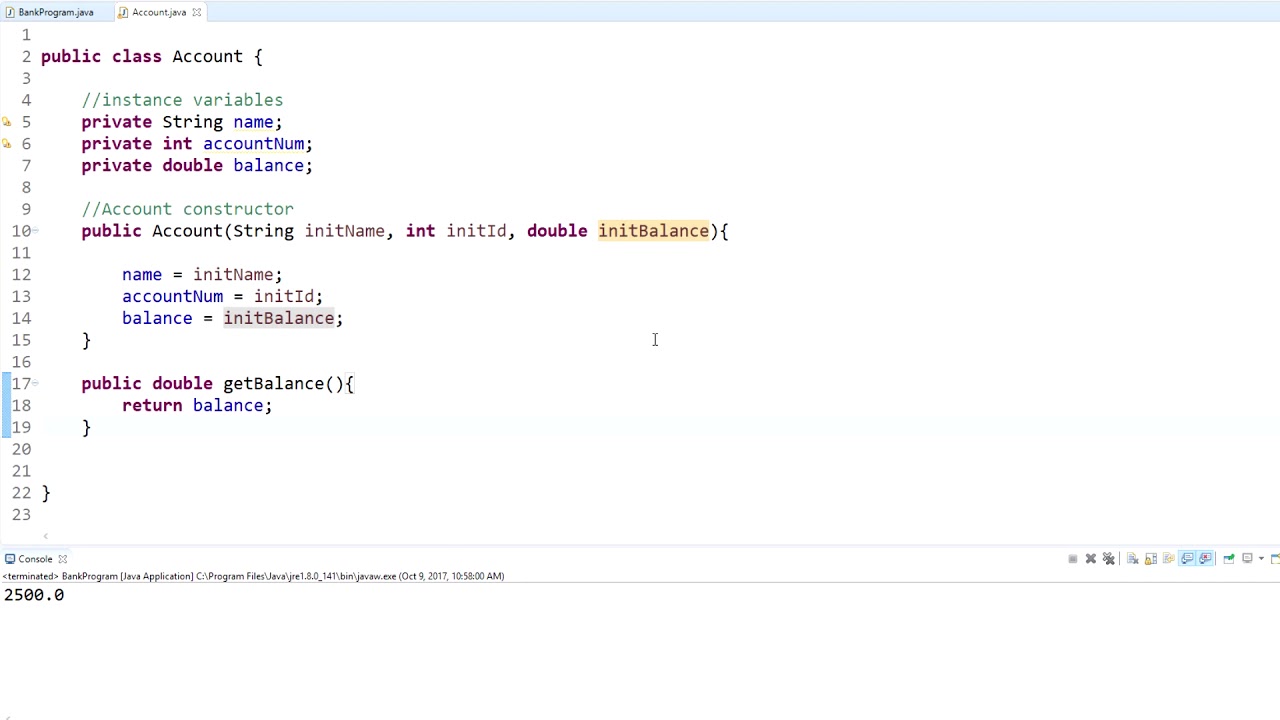
Java Programming Tutorial 15 Creating Instantiating Objects Youtube
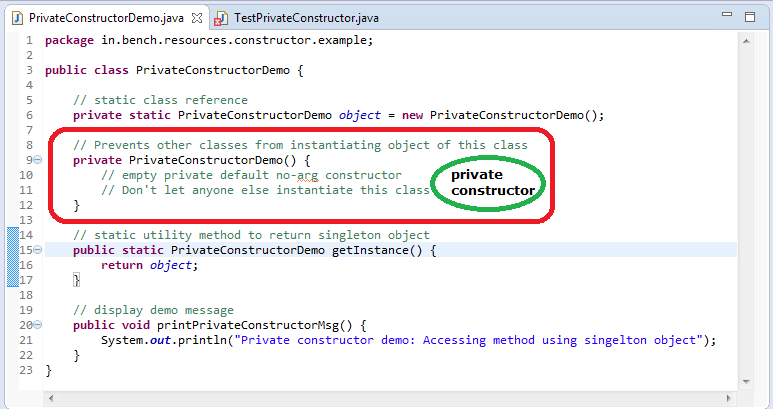
Java Private Constructor Benchresources Net

Java Debugger Find Where An Object Was Instantiated Stack Overflow

Call Java Methods With Dataweave Apisero

Class

Media Won T Instantiate Despite Correct Import Packages In Java Stack Overflow
Cannot Instantiate Remote Class Issue 49 Oracle Visualvm Github
Q Tbn 3aand9gcqwzig4dnlb3i0tzdytjilcbd3 Zudkhsxhs7h6uiihrlyziqko Usqp Cau

Tip Java Instantiating Generic Type Parameter Geekycoder